Using the powerGate .NET library
Follow these steps to use the powerGate library in your C# project
With the installation of powerGate on your development machine, the required .NET library will be installed for all users.
It contains the relevant API’s to communicate with an ERP System via OData.
The powerGate .NET library requires your project to target at least .NET Framework v4.7 or .NET 8!
1. Reference the powerGate.Erp.Client assembly
In Visual Studio right-click on References and click “Add References”.
Search for the assembly “powerGate.Erp.Client” in Assemblies-tab and add it to your project.
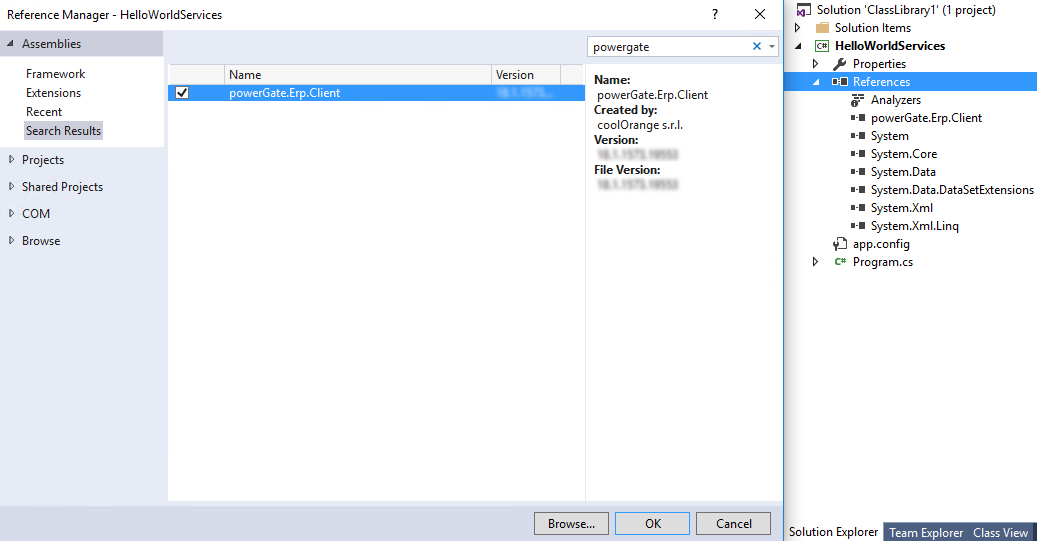
For .NET Framework projects the assembly will be referenced from the GAC; for .NET 8 projects, please reference it directly from C:\Program Files\coolOrange\Modules\powerGate.
In both cases, “Copy Local” should be set to “false”.
Note: This requires separate resolving routines for .NET 8 applications (e.g. via Assembly.LoadFrom(…)).
2. Create an ErpClient instance and connect to a service
In order to gain access to the powerGate API’s, the following namespace must be imported:
using powerGate.Erp.Client;
Root entry point is the class ErpClient:
var client = new ErpClient();
Call the ConnectErp() method in order to have access to the prefered Service:
var northwindService = client.ConnectErp(new Uri("http://services.odata.org/V4/Northwind/Northwind.svc/"));
3. Communicate with the service
If we have successfully connected to a Service (service.Available will return true), then we are able to make CRUD operations on the prefered EntitySet.
See the following example with a single get, which retrieves exactly one entry:
var categories = client.Services["Northwind.svc"].EntitySets["Categories"];
var categoryKeys = new Dictionary<string,object> { {"CategoryID", 3} };
var category = categories.GetErpObject(categoryKeys);
Console.WriteLine("Successful retrieved category '{0}' with Id '{1}'.",
category["CategoryName"], (int)category["CategoryID"]);
4. Release the service when done
When you are done working with your ERP System, the ErpClient should be disposed, in order to disconnect from all the connected services and release all the resources.
client.Dispose();
We recommend using the “using” statement on the ErpClient, so Dispose() will be called in each situation, also when unexpected exceptions are thrown!
Install powerGate on customer machine
When shipping your projects binaries to your customer, also the customers machine requires a powerGate installation.
Therefore delivering the powerGate.Erp.Client assembly within your project should be avoided, in order to not lose the benefits from powerGates Update strategy.
See the complete example:
using System;
using System.Collections.Generic;
using powerGate.Erp.Client;
namespace HelloWorldServices
{
class Program
{
static void Main(string[] args)
{
using (var client = new ErpClient())
{
var northwindService = client.ConnectErp(new Uri("http://services.odata.org/V4/Northwind/Northwind.svc/"));
var categories = client.Services["Northwind.svc"].EntitySets["Categories"];
var categoryKeys = new Dictionary<string, object>{ {"CategoryID", 3} };
var category = categories.GetErpObject(categoryKeys);
Console.WriteLine("Successful retrieved category '{0}' with Id '{1}'.", category["CategoryName"], (int)category["CategoryID"]);
}
Console.ReadLine();
}
}
}