Add-VaultTab
Adding a new Tab for a specific entity type to the Vault Explorer with an Action that is called when the Tab is clicked or the selection changes in Vault.
The cmdlet provides the possibility to define a user interface for the registered Tab.
Syntax
Add-VaultTab -Name <String> -EntityType <VaultTabEntityType> -Action <Scriptblock | String> [<CommonParameters>]
Parameters
Type |
Name |
Description |
Default Value |
Optional |
---|---|---|---|---|
String |
Name |
Label of the Tab displayed in the Vault Explorer. |
no |
|
VaultTabEntityType |
EntityType |
The entity type for which the Tab should be displayed. |
no |
|
Scriptblock / String |
Action |
Script block or function that gets executed for the currently selected entity in the Vault Client ( |
no |
Return type
empty
Remarks
The Cmdlet extends the Vault Client’s user interface with a new tab with the specified -Name as its label.
When multiple tabs with the same -Name for the same -EntityType are registered, only the latest registered tab is displayed.
The script block or the function name passed as -Action parameter is invoked for the element that is selected in the Vault Client.
This can be a powerVault File, a Folder, an Item or a Custom Object, depending on the specified -EntityType.
The Action runs whenever the user activates the tab, or selects a different element while the tab is visible.
If multiple elements are selected, the first selected entity will be used.
In case the Action returns an invalid WPF Control or if an unhandled terminating exception occurs in the Vault Client’s UI thread, the tab content is cleared and details are logged.
Note
The cmdlet can only be used when running directly in a Vault Explorer process.
Created tabs remain available as long as this process exists and disappear again when the Vault Client is restarted.
Only the use of the cmdlet in client customization scripts ensures that the tab is displayed in every Vault Client session.
The Vault Client remembers activated Tabs (based on their position) even after a restart. In this case, the passed -Action gets invoked immediately after LoginVault event registrations.
Examples
Adds a tab for Files displaying a DataGrid with their FileBom rows:
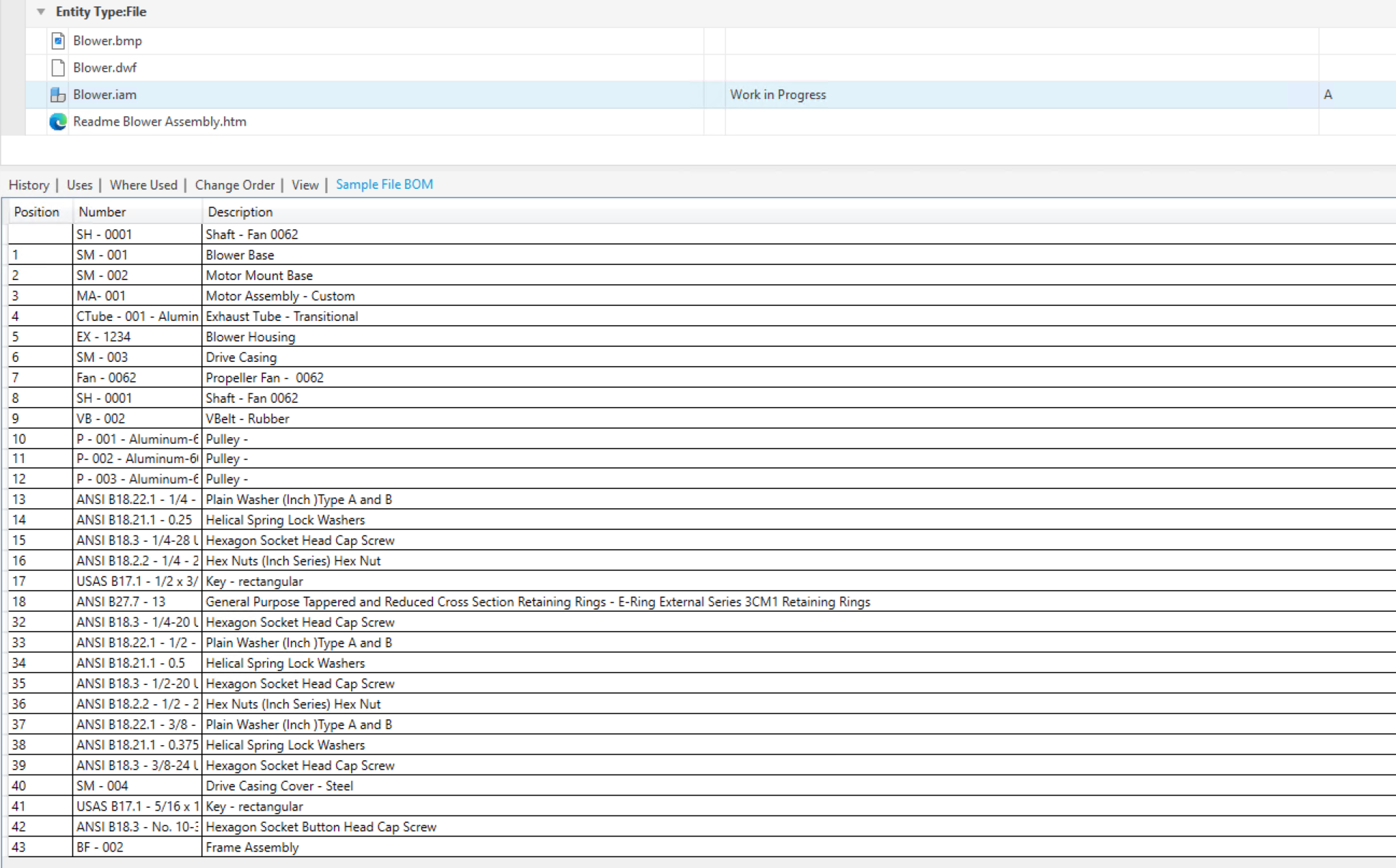
Add-VaultTab -EntityType File -Name 'Sample File BOM' -Action {
param($selectedFile)
$fileBom = Get-VaultFileBOM -File $selectedFile._FullPath
if(-not $fileBom) {
return BuildFromXaml @'
<StackPanel xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation">
<Label Content="There are no rows to show in this view." HorizontalAlignment="Center" />
</StackPanel>
'@
}
#Print BOM data as Table like in Inventor
$bomrows_table = BuildFromXaml @'
<DataGrid xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation" AutoGenerateColumns="False" IsReadOnly="True">
<DataGrid.Columns>
<DataGridTextColumn Header="Position" Binding="{Binding Bom_PositionNumber}" Width="60" />
<DataGridTextColumn Header="Number" Binding="{Binding Bom_Part Number}" Width="120" />
<DataGridTextColumn Header="Description" Binding="{Binding Description}" Width="*" />
<DataGridTextColumn Header="Quantity" Binding="{Binding Bom_Quantity}" Width="60" />
<DataGridTextColumn Header="Unit of Measure" Binding="{Binding Bom_Unit}" Width="60"/>
</DataGrid.Columns>
</DataGrid>
'@
$bomrows_table.ItemsSource = @($fileBom | sort-object {[int]$_.Bom_PositionNumber})
return $bomrows_table
}
function BuildFromXaml([xml]$xaml){
$xamlReader = New-Object System.Xml.XmlNodeReader $xaml
return [Windows.Markup.XamlReader]::Load($xamlReader)
}
Adds a tab for Items that displays their Thumbnail image:
Add-VaultTab -EntityType Item -Name 'Thumbnail' -Action 'DisplayItemThumbnail'
function DisplayItemThumbnail($selectedItem){
$imageControl = New-Object System.Windows.Controls.Image
$imageControl.Source = $selectedItem._Thumbnail.Image
return $imageControl
}
Adds a tab for Folders to display information from Fusion Lifecycle using powerPLM (powerFLC):
#Connect-FLC
Add-VaultTab -EntityType Folder -Name 'Fusion Lifecycle' -Action {
param($selectedFolder)
$flcItem = Get-FLCItems -Workspace 'Products' -Filter "ITEM_DETAILS:NUMBER=$($selectedFolder._Name)" -ErrorAction Continue
$tab_control = [Windows.Markup.XamlReader]::Load([Xml.XmlNodeReader]::new([xml] @"
<Grid xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation" xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml">
$(
if($global:flcConnection) {
"<TextBlock Text=""Connected to Tenant: $($flcConnection.Tenant) - User: $($flcConnection.UserId) - Workspace: Products"" />"
} else {
"<TextBlock Text=""$($Error[0])"" FontWeight=""Bold"" Foreground=""Red"" />"
}
)
<StackPanel Orientation="Horizontal" Grid.Row="1">
$(
if($flcItem) {
'<TextBlock Text="The selected folder was created through FLC synchronization:" />'
} else {
'<TextBlock Text="The selected folder was not created through FLC synchronization." FontWeight="Bold" Grid.Row="1" />'
}
)
<TextBlock Margin="10,0,0,0"><Hyperlink x:Name="OpenInFLCHyperlink">$($flcItem.Number) $($flcItem.Description)</Hyperlink></TextBlock>
</StackPanel>
<Button x:Name="SyncFLCButton" Content="Sync with Fusion Lifecycle ..." Width="200" Height="30" Grid.Column="1" Grid.Row="1" />
<Grid.ColumnDefinitions>
<ColumnDefinition Width="Auto" />
<ColumnDefinition Width="*" />
</Grid.ColumnDefinitions>
<Grid.RowDefinitions>
<RowDefinition Height="30" />
<RowDefinition Height="auto" />
</Grid.RowDefinitions>
</Grid>
"@))
$tab_control.FindName('OpenInFLCHyperlink').Add_Click({
$navigationUrl = "$($flcConnection.Url)plm/workspaces/$($flcConnection.Workspaces.Find($flcItem.Workspace).Id)/items/itemDetails?view=split&itemId=urn%60adsk,plm%60tenant,workspace,item%60$($flcConnection.Tenant.ToUpper()),$($flcConnection.Workspaces.Find($flcItem.Workspace).Id),$($flcItem.Id)"
Start-Process $navigationUrl
}.GetNewClosure())
$tab_control.FindName('SyncFLCButton').Add_Click({
Add-VaultJob 'coolorange.flc.sync.folder' -Description 'This example requires the "New Product Introduction (NPI)" workflow job from https://github.com/coolOrangeLabs/powerflc-samples/tree/master/coolorange.flc.sync.folder'
})
return $tab_control
}
Adds a tab for the Custom Objects of type ‘Task’ to display information:
Add-VaultTab -EntityType Task -Name 'Properties' -Action {
param($selectedTask)
$tab_control = [Windows.Markup.XamlReader]::Load([Xml.XmlNodeReader]::new([xml] @'
<Grid xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation" xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml">
<Grid.ColumnDefinitions>
<ColumnDefinition Width="Auto" />
<ColumnDefinition Width="*" />
</Grid.ColumnDefinitions>
<Grid.RowDefinitions>
<RowDefinition Height="Auto" />
<RowDefinition Height="Auto" />
<RowDefinition Height="Auto" />
<RowDefinition Height="Auto" />
<RowDefinition Height="Auto" />
</Grid.RowDefinitions>
<Label Grid.Column="0" Grid.Row="0" Content="Category"/>
<TextBox Grid.Column="1" Grid.Row="0" Text="{Binding _CategoryName}" />
<Label Grid.Column="0" Grid.Row="1" Content="Name"/>
<TextBox Grid.Column="1" Grid.Row="1" Text="{Binding _Name}" />
<Label Grid.Column="0" Grid.Row="2" Content="State"/>
<TextBox Grid.Column="1" Grid.Row="2" Text="{Binding _State}" />
<Label Grid.Column="0" Grid.Row="3" Content="Create Date"/>
<TextBox Grid.Column="1" Grid.Row="3" Text="{Binding _CreateDate}" />
<Label Grid.Column="0" Grid.Row="4" Content="Created By"/>
<TextBox Grid.Column="1" Grid.Row="4" Text="{Binding _CreateUserName}" />
</Grid>
'@))
$tab_control.DataContext = $selectedTask
return $tab_control
}